Redirect Laravel Route Externally Including Query Parameters
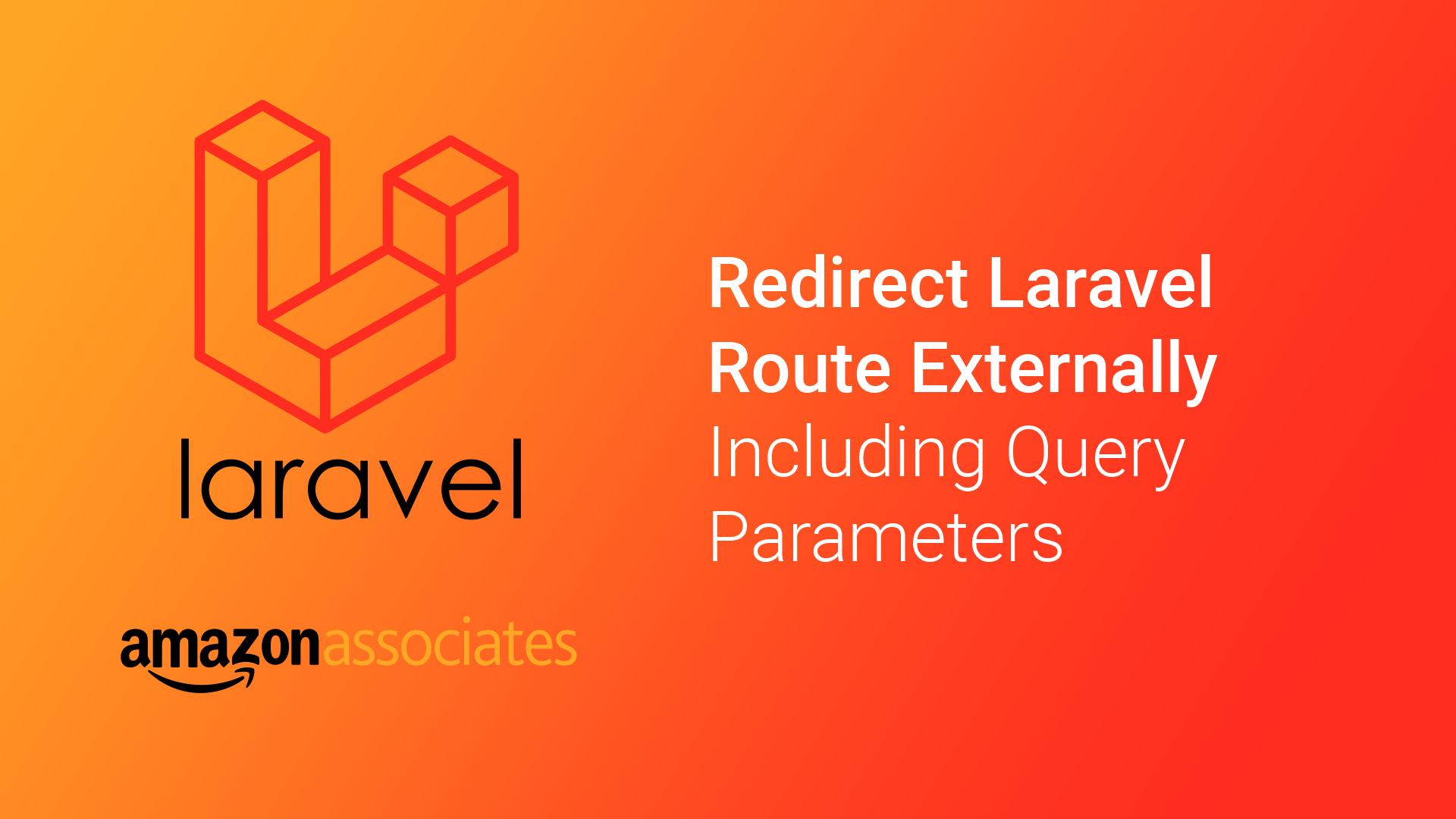
Introduction
We use affiliate marketing as a source of revenue on this website. In order to earn a commission, we need to include our affiliate tag in every affiliate link. If we hardcode the link or the affiliate tag and either of those changes, we would have to go back and update them on every page. A better solution is to reference a programmatically generated link.
Generating Affiliate Links Programmatically
Goals
Here were our design goals:
Easy to use
Easy to update if the URL or affiliate tag changes
Ability to link directly to products or searches on the affiliate website
Reusable for other affiliate programs in the future
Implementation
// Redirect "/go/amazon*" to Amazon and include the Amazon Associates tag
Route::get('/go/amazon{segments?}',
function (Request $request, String $segments = null) {
// Get the Amazon environment variables
$amazonUrl = env('AMAZON_URL');
$amazonTag = env('AMAZON_TAG');
// Construct the Amazon Associates tag parameter
$amazonParam = ['tag' => $amazonTag];
// Construct the base url from the Amazon URL and segments passed
$baseUrl = $amazonUrl . $segments;
// Get the query parameters
$query = $request->query();
// Merge any existing query parameters with the Amazon Associates tag parameter
$newQuery = array_merge($query, $amazonParam);
// Construct the new URL
$newUrl = $baseUrl . '?' . http_build_query($newQuery);
// Redirect to the new URL
return Redirect::away($newUrl);
// Include all the segments
})->where('segments', '.*');
Here are the corresponding environment variables stored in .env
:
AMAZON_URL=https://www.amazon.com
AMAZON_TAG=bitesizebyt0c-20
Design Notes
By using environment variables
AMAZON_URL
andAMAZON_TAG
, we can update them if necessary.By using redirects:
The redirect can be generated programmatically.
The browser will make 2 requests – one request to the current website and then another request to the affiliate website.
By combining the optional segments
({segments?})
and thewhere('segments', '.*') clause
, the code handles linking to items on the affiliate website.By combining the existing query parameters with the affiliate tag, the code handles searches on the affiliate website.
This code could be modified for use with other affiliate programs by matching the second segment.
Conclusion
By combining environment variables, redirection, optional segments, and the where
clause, we were able to meet our design goals.
Software Versions
PHP 8.1.8
Laravel 8.19.0
References:
-
Optional segments in Laravel routes with an optional sub-path on Stack Overflow
-
Where clause in Laravel - Using (:any?) wildcard for ALL routes? on Stack Overflow
-
Merging arrays in PHP append one array to another (not array_push or +) on Stack Overflow
-
Route::away in Redirecting To External Domains in Laravel 9 Documentation
-
Building query strings in http_build_query in PHP Documentation
-
Amazon Associates tracking ID in Amazon Associates Affiliate Program - Manage Your Tracking ID
-
"Programming PHP: Creating Dynamic Web Pages 4th Edition" by Kevin Tatroe on Amazon
-
"Laravel: Up & Running: A Framework for Building Modern PHP Apps (2nd Edition)" by Matt Stauffer on Amazon